Section 5.7 While Loops Sentinel
Subgoals for Evaluating a Loop.
-
Determine Loop Components
- Start condition and values
- Iteration variable and/or update condition
- Termination/final condition
- Body that is repeated based on indentation
- Trace the loop, writing updated values for every iteration or until you identify the pattern
Subsection 5.7.1
Problem: Given the following code, what is the output if the user enters the values: 10, 15, 20, 25, 30, 35, -1?
print("Enter a negative score to signal the end of input.")
good_scores = 0
score = int(input("Score: "))
while score >= 0:
if score >= 20:
good_scores += 1
score = int(input("Score: "))
print("Number of good scores: ", good_scores)
Subsection 5.7.2 Determine loop components
Starting values:
good_scores = 0
# score will be the integer 10 since that is the first value the user entered
Termination condition: When score is greater than or equal to 0
Body that is repeated based on indentation:
if score >= 20:
good_scores += 1
score = int(input("Score: "))
Subsection 5.7.3 Trace the loop, writing updated values for every iteration or until you identify the pattern
For every iteration of loop, write down values.
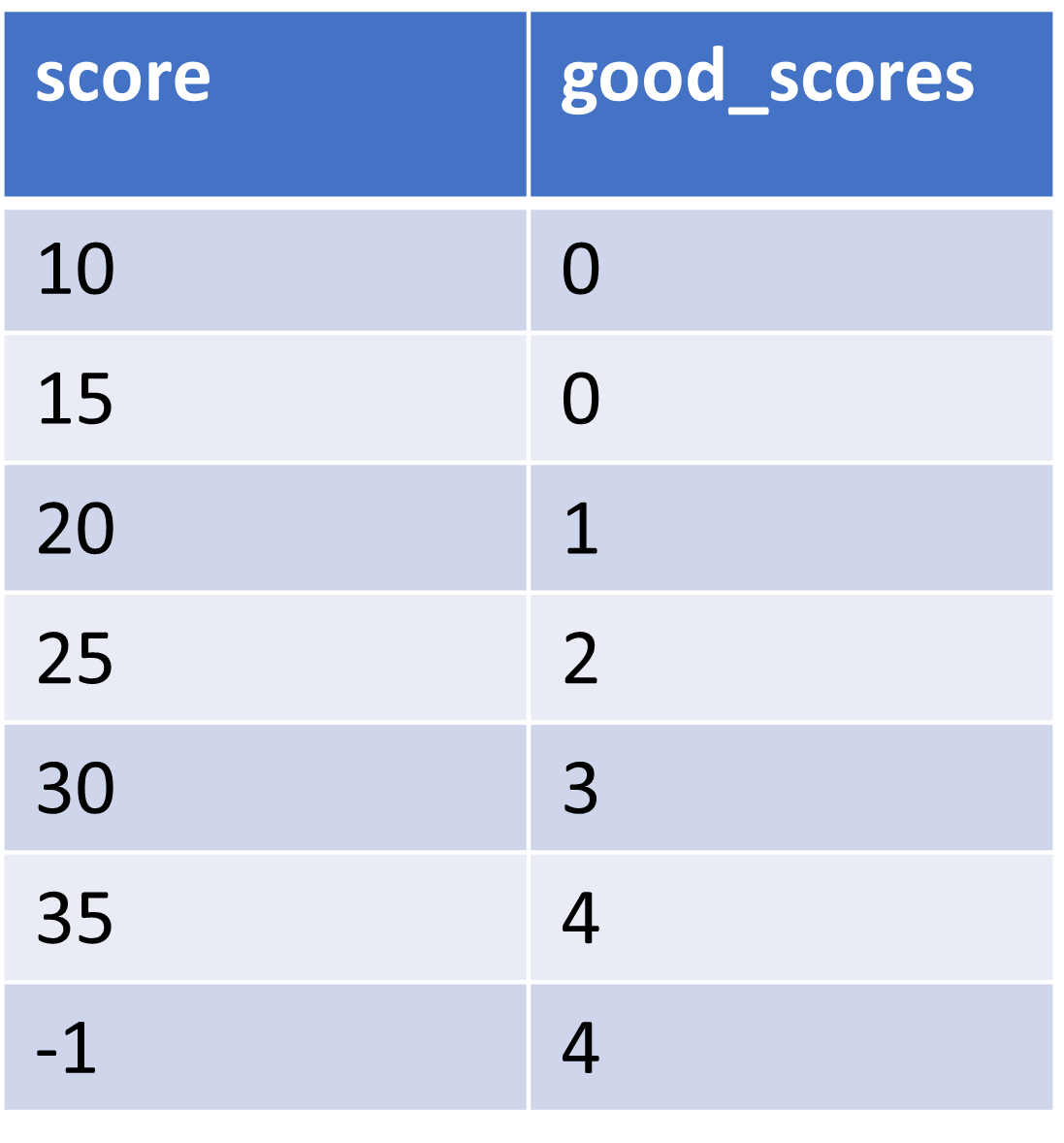
Number of good scores: 4
You have attempted of activities on this page.