Section 5.5 Complex Conditional
Subgoals for Evaluating a Loop.
-
Determine Loop Components
- Start condition and values
- Iteration variable and/or update condition
- Termination/final condition
- Body that is repeated based on indentation
- Trace the loop, writing updated values for every iteration or until you identify the pattern
Subsection 5.5.1
Problem: Given the following code, what is the output if the user enters 50, 70, 80, 65, 90, 100, 0, 5, 102?
print("Enter a value. An invalid value will end input.")
passing = 0
failing = 0
value = int(input("Score: "))
while value >= 0 and value <= 100:
if value >= 70:
passing+= 1
else:
failing+= 1
value = int(input("Score: "))
print("Total number of scores:", passing+failing)
print("Passing:", passing, " Failing:", failing)
Subsection 5.5.2 Determine loop components
Starting values:
passing = 0
failing = 0
# value will be the 50 since that is the first value entered by the user
Termination condition: When value is between 0 and 100 (inclusive)
Body that is repeated based on indentation:
if value >= 70:
passing+= 1
else:
failing+= 1
value = int(input("Score: "))
Subsection 5.5.3 Trace the loop, writing updated values for every iteration or until you identify the pattern
For every iteration of loop, write down values.
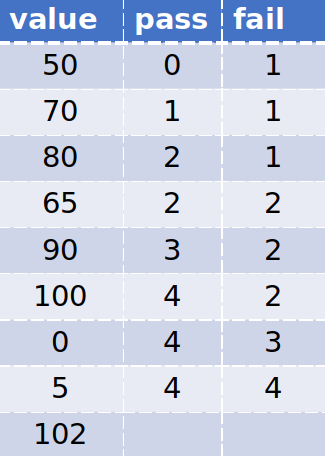
Answer.
Total number of scores: 8 Passing: 4 Failing: 4
You have attempted of activities on this page.