Section 5.11 Nested Loops
Subgoals for Evaluating a Loop.
-
Determine Loop Components
- Start condition and values
- Iteration variable and/or update condition
- Termination/final condition
- Body that is repeated based on indentation
- Trace the loop, writing updated values for every iteration or until you identify the pattern
Subsection 5.11.1
Problem: Given the following code, what is the output?
i = 0
j = 0
m = 8
n = 5
while i < n:
j = 0
while j < m:
print("*", end="")
j += 1
print()
i += 1
Subsection 5.11.2 Determine loop components
Starting values:
i = 0
j = 0
m = 8
n = 5
Termination condition: When i is greater than n
Body that is repeated based on indentation:
j = 0
while j < m:
print("*", end="")
j += 1
print()
i += 1
Subsection 5.11.3 SG2: Trace the loop, writing updated values for every iteration or until you identify the pattern
Think of an analog clock:
Second hand goes completely around before minute hand moves
Just like inner loop:
Inner loop must finish before outer loop increments once
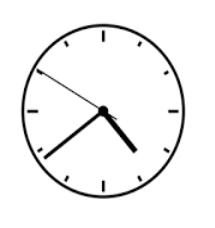
Looking at ONLY the outer loop: <NL> is a new line
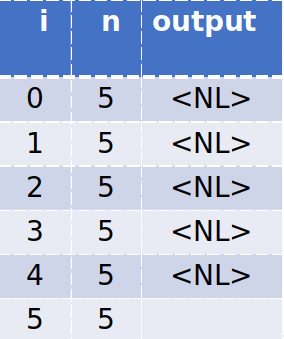
Now Repeat for inner loop
Subsection 5.11.4 SG1: Determine loop components
Starting values:
j = 0
m = 8
Termination condition: When j is less than m.
Body that is repeated based on indentation:
print("*", end="")
j += 1
Subsection 5.11.5 SG2: Trace the loop, writing updated values for every iteration or until you identify the pattern
Looking at Only the inner loop:
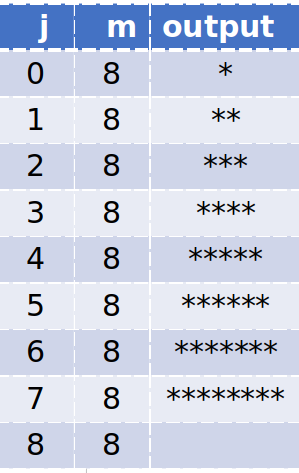
Remember, this was just for the first iteration of the outer loop, while i is still value 0.
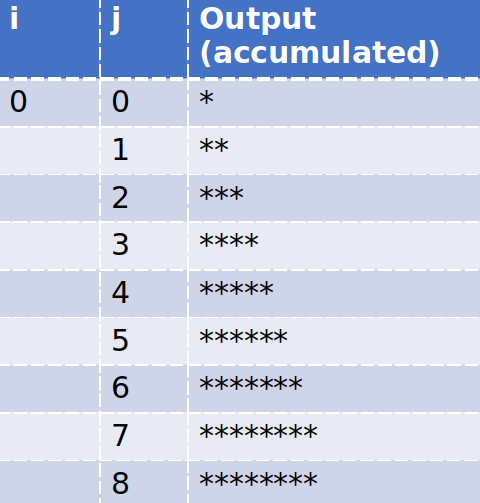
Continuing to the next iteration of the outer loop, i is 1 and j is re-started at value 0:
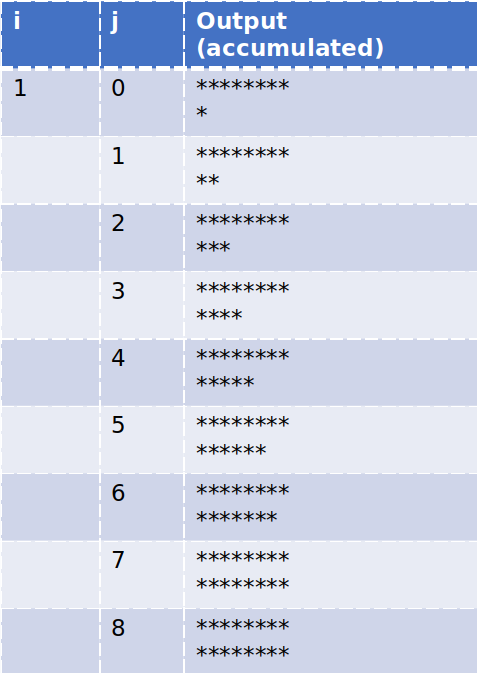
Again, increment i to 2, and j is re-started at 0:
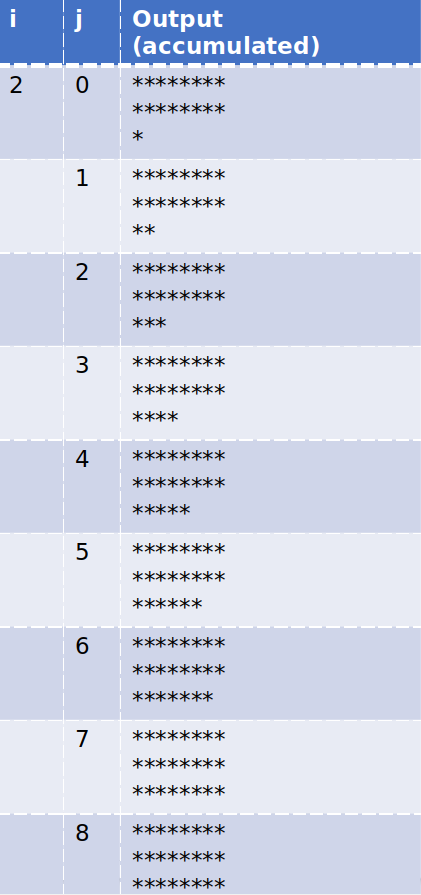
Note that outer loop goes 5 times,
Inner loop goes 8 times (for each outer loop)
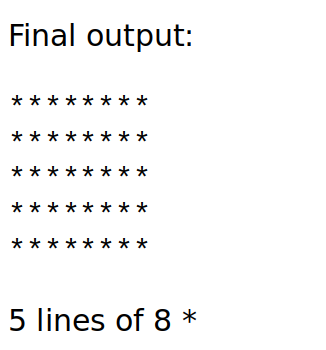
The final output will be 5 lines of 8 asterisks (*).
You have attempted of activities on this page.