Section 8.1 Variables Call
Subgoals for evaluating a function call:.
- Create stack frame for global variables
- Ensure function is defined before function call before beginning body of function
- Determine parameter values based on positional arguments, keyword arguments, and default values to initialize local variables
- Use stack frame for function to trace local variables
- At return to call site, pass values back to call site
- Delete stack frame with local variables after return to call site
Subsection 8.1.1
Given the following declarations
def foo(alpha, beta):
delta = alpha * beta - gamma
return delta * 2
alpha = 9
gamma = 1
Evaluate these statements and determine the final value of the top-level variables.
kappa = foo(7, alpha) - 20
Subsection 8.1.2 1. Create stack frame for global variables
Prior to the execution of the function, there are two global variables (
alpha
and gamma
). The variable kappa
will not be defined until after the function foo
is executed, so for now our stack frame only has two variables.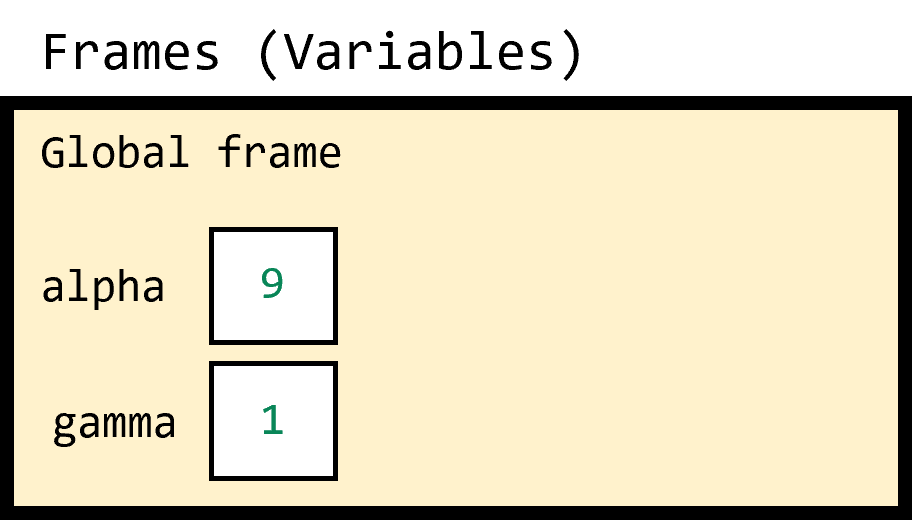
Subsection 8.1.3 2. Ensure function is defined before function call before beginning body of function
The function
foo
was defined at the very start of the program, and foo
is being called at the very end. Therefore, it is safe to say that the function is defined before it was called.Subsection 8.1.4 3. Determine parameter values based on positional arguments, keyword arguments, and default values to initialize local variables
When we execute
foo
, we must match each provided argument with the corresponding parameters.- The first argument
7
is matched to the parameteralpha
- The second argument
alpha
is substituted for9
(the value associated with that variable in the Global frame), and then9
is matched to the parameterbeta
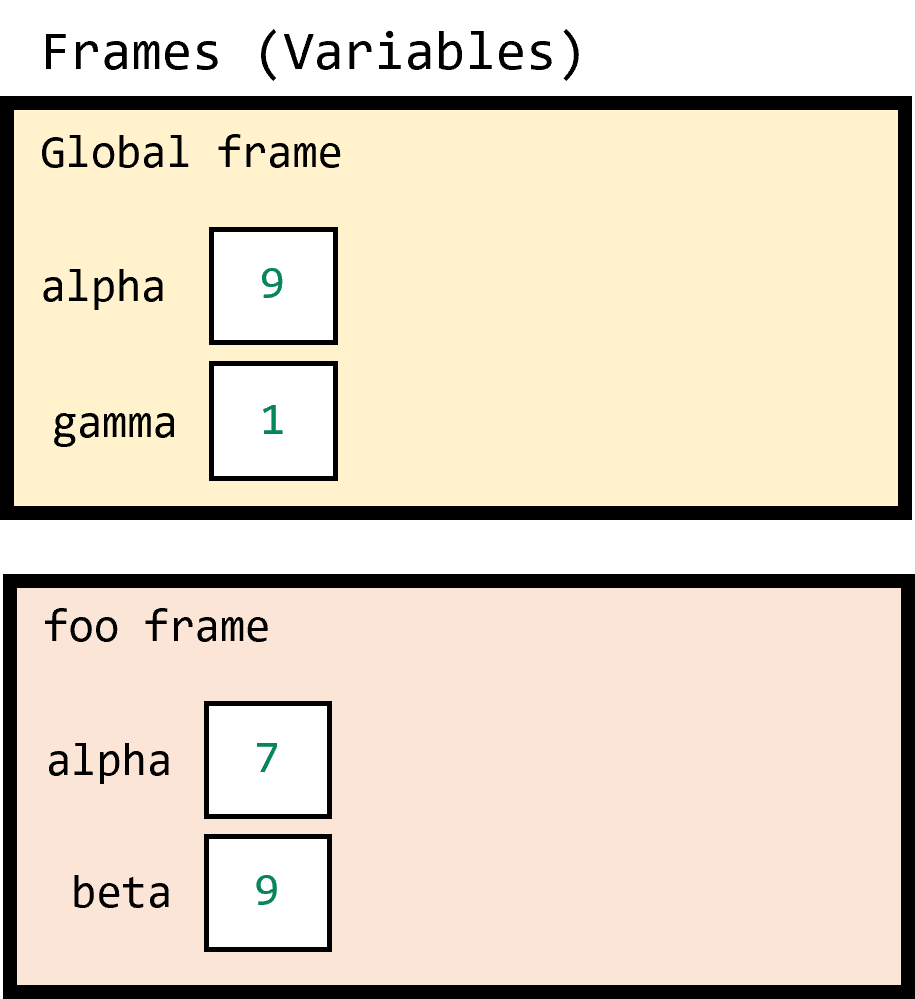
Notice that the global variable
alpha
is completely distinct from the parameter alpha
inside the function foo
frame. The two variables have the same name, but they are in different frames so they each have their own separate values.Subsection 8.1.5 4. Use stack frame for function to trace local variables
We begin executing the body of the function, one line at a time.
delta = alpha * beta - gamma
In the first line, we evaluate the right-hand side first, substituting the appropriate values:
- The
alpha
is substituted for thealpha
variable local to thefoo
frame, which has the value9
currently. - The
beta
is substituted for thebeta
variable local to thefoo
frame, which has the value9
currently. - The
gamma
is substituted for thegamma
variable from the global frame (since there are no local variables with that name), which has the value1
currently.
The expression becomes
7 * 9 - 1
, which is 62
. The value 62
is assigned to a new local variable delta
.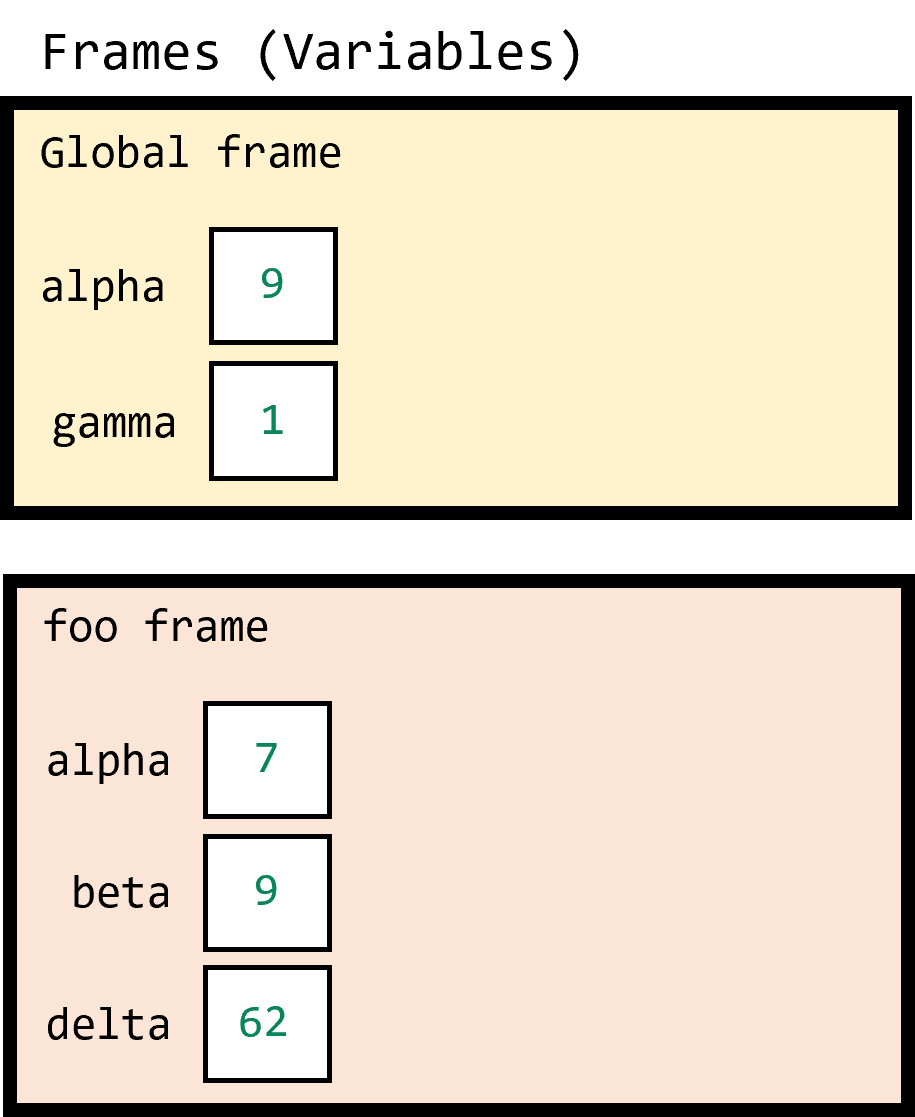
After we execute the first line of the body, we execute the second line, which is a
return
statement.return delta * 2
In that line of code, we substitute
62
for delta
and then multiply the result by 2
. The final result is 124
.Subsection 8.1.6 5. At return to call site, pass values back to call site
The original function call to
foo
is now complete, with the value 124
to be returned. We substitute the foo(7, alpha)
expression for 124
.Subsection 8.1.7 6. Delete stack frame with local variables after return to call site
Because the function call is completed, we must delete the stack frame. The variables that existed inside the stack frame are not considered among the final global variables.
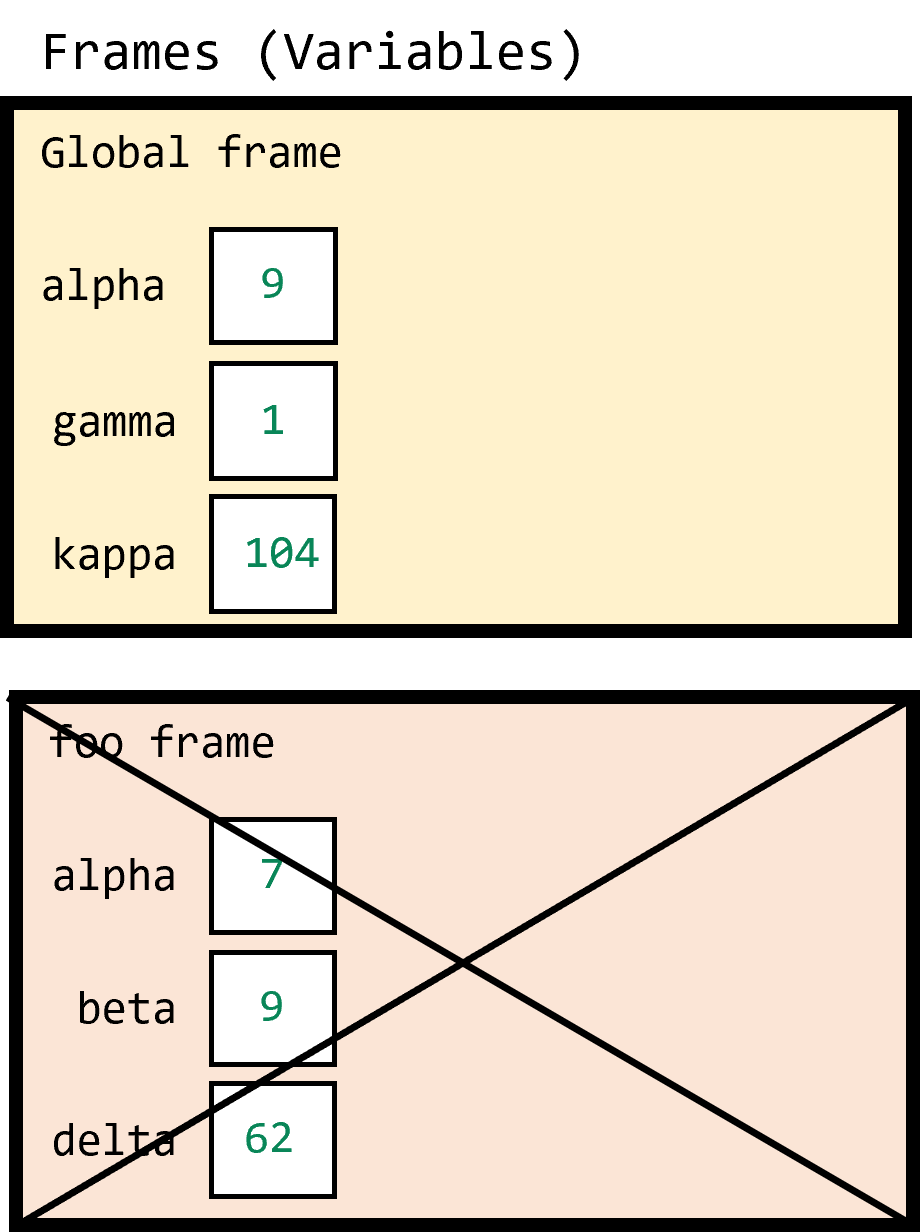
We can now finish executing the original right-hand side of the expression:
124 - 20
is 104
, so the final result 104
is assigned to kappa
.Subsection 8.1.8 Answer
alpha
:9
gamma
:1
kappa
:104
These are the only variables in the global frame, since the other stack frame was deleted.
You have attempted of activities on this page.