5.5. Mutators / Setters¶
As we saw in the last section, since we typically make instance variables
private
, we have to define getters if we want to allow code outside the
class to access the value of particular instance variables.
By the same token, if we want to allow code outside the class to change the
value of an instance variable we have to provide what is formally called a
mutator method but which everyone actually calls a setter. A setter is a
void method with a name that starts with set
and that takes a single
argument of the same type as the instance variable to be set. The effect of a
setter, as you would probably expect, is to assign the provided value to the
instance variable.
Just as you shouldn’t reflexively write a getter for every instance variable, you should think even harder about whether you want to write a setter. Not all instance variables are meant to be manipulated directly by code outside the class.
For example, consider the Turtle
class. It provides getters getXPos
and
getYPos
but it does not provide corresponding setters. There are, however,
methods that change a Turtle
’s position like forward
and moveTo
.
But they do more than just changing the values of instance variables; they also
take care of drawing lines on the screen if the pen is down. By not providing
setters for those instance variables, the authors of the Turtle
class can
assume the a Turtle
’s position won’t change other than by going through
one of the approved movement methods. In general, you shouldn’t write a setter
until you find a real reason to do so.
5.5.1. How to write a setter¶
Here are some examples of how to write a setter for an instance variable:
class ExampleTemplate
{
// Instance variable declaration
private typeOfVar varName;
// Setter method template
public void setVarName(typeOfVar varName)
{
this.varName = varName;
}
}
As with constructors, setters often use the idiom of using the same name for the
paramater as the name of the instance variable that is being set and then using
this.name
to explicitly refer to the instance variable when setting it.
Here’s an example of the Student
class with a setter for the name
variable:
class Student
{
// Instance variable name
private String name;
/**
* setName sets name to newName
*
* @param name
*/
public void setName(String name)
{
this.name = name;
}
public static void main(String[] args)
{
// To call a set method, use objectName.setVar(newValue)
Student s = new Student();
s.setName("Ayanna");
}
}
Notice the difference between setters and getters in the following figure.
Getters return an instance variable’s value and have the same return type as the
variable and no parameters. Setters have a void
return type and a single
parameter of the same type as the instance variable whose value is assigned to
the instance variable by the setter.
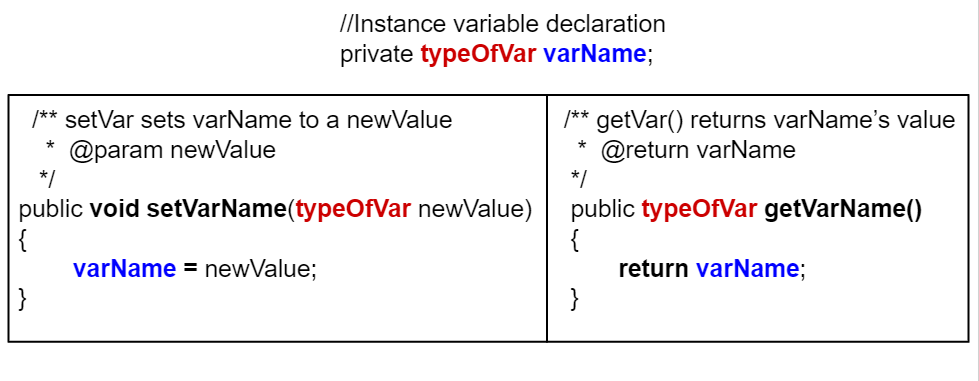
Figure 1: Comparison of setters and getters¶
Try the Student
class below which has had some setters added. Notice that
there is no setId
method even though there is a getId
. This is
presumably because in the system this class is part of, while it makes sense for
a student to change their name or email, their id should never change.
You will need to fix one error. The main
method is in a separate class
TesterClass
and does not have access to the private
instance variables
in the `Student
class. Change the main
method so that it uses a
public
setter to change the value instead.
Fix the main method to include a call to the appropriate set method.
public int getPeople(int people)
-
The set method should not have a return value and is usually named set, not get.
public int setPeople()
-
The set method should not have a return value and needs a parameter.
public int setPeople(int people)
-
The set method should not have a return value.
public void setPeople(int people)
-
Yes, the set method should take a parameter called people and have a void return value. The name of the set method is usually set followed by the full instance variable name, but it does not have to be an exact match.
public int setPeople(int p)
-
The parameter of this set method should be called people in order to match the code in the method body.
5-5-2: Consider the class Party which keeps track of the number of people at the party.
public class Party
{
// number of people at the party
private int people;
/* Missing header of set method */
{
this.people = people;
}
}
Which of the following method signatures could replace the missing header for the set method in the code above so that the method will work as intended?
-
5-5-3: Drag the definition from the left and drop it on the correct word on the right. Click the "Check Me" button to see if you are correct.
Review the vocabulary.
- gets and returns the value of an instance variable
- accessor method
- sets the instance variable to a value in its parameter
- mutator method
- initializes the instance variables to values
- constructor
- accessible from outside the class
- public
- accessible only inside the class
- private
Mutator methods do not have to have a name with “set” in it, although most do. They can be any methods that change the value of an instance variable or a static variable in the class, as can be seen in the AP Practice questions below.
5.5.2.
Programming Challenge : Class Pet Setters¶
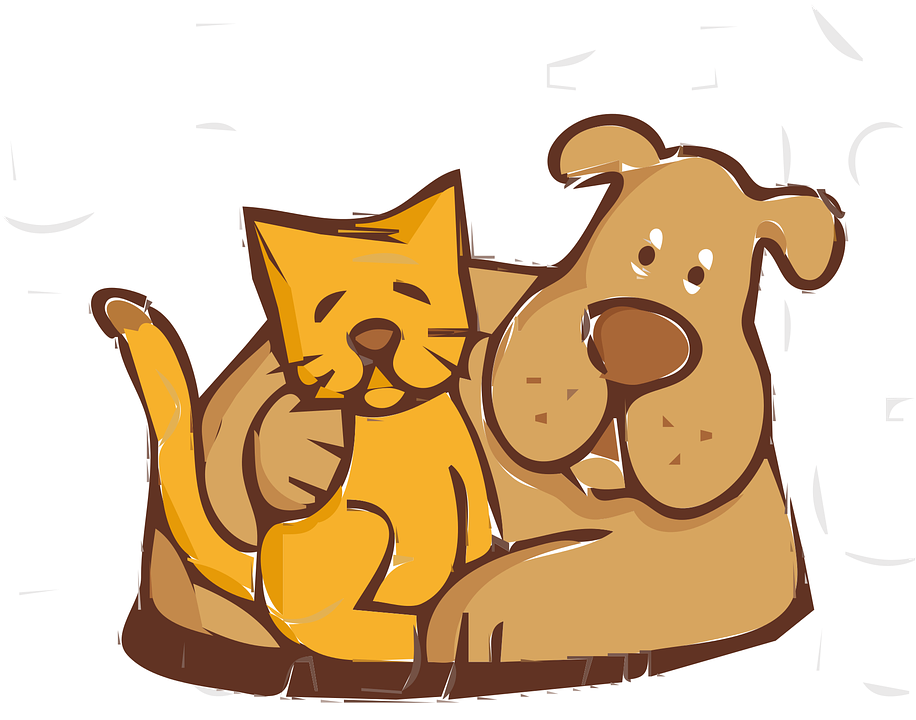
Copy your Awesome Animal Clinic Pet class from the last lesson into this Active Code window.
Add set methods for each of the 5 instance variables. Make sure you use good commenting!
Test each of the set methods in the main method.
Create a Pet
class that keeps track of the name, age, weight, type of
animal, and breed for records at an animal clinic with a constructor, a
toString
method, and getters and setters for each instance variable.
5.5.3. Summary¶
A void method does not return a value. Its header contains the keyword
void
before the method name.A mutator method or setter is a void method that changes the values of an instance or static variable.
5.5.4. AP Practice¶
The constructor header does not have a return type.
-
The constructor should not have a return type.
The resetTemp method is missing a return type.
-
Mutator methods usually have a void return type.
The constructor should not have a parameter.
-
Constructors can have parameters.
The resetTemp method should have a parameter.
-
Correct! The resetTemp method should have a parameter for the newTemp value to set the currentTemp.
The instance variable currentTemp should be public instead of private.
-
Instance variables should be private variables.
5-5-5: Consider the following class definition.
public class Liquid
{
private int currentTemp;
public Liquid(int temp)
{
currentTemp = temp;
}
public void resetTemp()
{
currentTemp = newTemp;
}
}
Which of the following best identifies the reason the class does not compile?
- Replace line 12 with numOfPeople = additionalPeople;
- This method should add additionalPeople to numOfPeople.
- Replace line 12 with return additionalPeople;
- This method should add additionalPeople to numOfPeople.
- Replace line 12 with additionalPeople += 3;
- This method should add additionalPeople to numOfPeople.
- Replace line 10 with public addPeople (int additionalPeople)
- Mutator methods should have a void return type.
- Replace line 10 with public void addPeople(int additionalPeople)
- Mutator methods should have a void return type.
5-5-6: In the Party
class below, the addPeople
method is intended to increase the value of the instance variable numOfPeople
by the value of the parameter additionalPeople
. The method does not work as intended.
public class Party
{
private int numOfPeople;
public Party(int n)
{
numOfPeople = n;
}
public int addPeople(int additionalPeople) // Line 10
{
numOfPeople += additionalPeople; // Line 12
}
}
Which of the following changes should be made so that the class definition compiles without error and the method addPeople
works as intended?