5.4. Accessors / Getters¶
Since the instance variables in a class are usually marked as private
to the
class, if you want code outside the class to be able to access the value of an
instance variable, you need to write what is formally called an accessor
methods but which everyone actually just calls a getter. A getter is a
public
method that takes no arguments and returns the value of the
private
instance variable. (We discussed using getters in section 2.5.)
If you used a language like App Inventor in an AP CSP class, you may have used setter and getter blocks. In App Inventor, you cannot make your own classes, but you can declare UI objects like Button1, Button2 from the Button class and use their get/set methods for any property like below. (We’ll talk about setters in Java in the next section.)
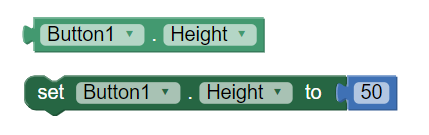
Figure 1: App Inventor Set and Get blocks for object Button1¶
You don’t need to write a getter for every instance variable in a class but if you want code outside the class to be able to get the value of one of your instance variables, you’ll need to write a getter that looks like the following.
class ExampleTemplate
{
// Instance variable declaration
private typeOfVar varName;
// Accessor (getter) method template
public typeOfVar getVarName()
{
return varName;
}
}
Notice that the getter’s return type is the same as the type of the instance
variable and all the body of the getter does is return the value of the variable
using a return
statement. (We’ll talk more about the return
statement in
section 5.6 but for now just notice that it is followed by an expression whose
value must be the same type as the return type in the method’s header. In a
getter that will definitely be true as long as the type of the instance variable
and the return type of the getter are the same.)
Here’s an example of an accessor method called getName
for the Student
class which also demonstrates how to call getName
using a Student
object:
class Student
{
//Instance variable name
private String name;
/** getName() example
* @return name */
public String getName()
{
return name;
}
public static void main(String[] args)
{
// To call a get method, use objectName.getVarName()
Student s = new Student();
System.out.println("Name: " + s.getName() );
}
Note, that getters only return the value of the variable. In other words, the
code that called the getter and which receives that value has no ability to
change the object’s instance variable; they just get a copy of the value.
However if the instance variable is a reference type like String
or
Person
the value that is copied is the value of the reference. That means
the caller receives a new copy of the reference that points to the same object
as is stored in the instance variable. In the next section, when we talk about
mutation, you’ll see how that means that the caller might be able to change the
object even though it can’t change the reference.
Note
Some common errors when writing and using getters are:
Forgetting a return type like
int
before the method name.Forgetting to use the
return
keyword to return a value at the end of the method.Forgetting to do something with the value returned from a method, like assigning it to a variable or printing it out.
Try the following code. Note that this active code window has 2 classes! The main method is in a separate Tester or Driver class. It does not have access to the private instance variables in the other Student class. Note that when you use multiple classes in an IDE, you usually put them in separate files, and you give the files the same name as the public class in them. In active code and IDEs, you can put 2 classes in 1 file, as demonstrated here, but only 1 of them can be public and have a main method in it. You can also view the fixed code in the Java visualizer.
Try the following code. Note that it has a bug! It tries to access the private instance variable email from outside the class Student. Change the main method in Tester class so that it uses the appropriate public accessor method (get method) to access the email value instead.
5.4.1. toString
¶
While not strictly speaking a getter, another important method that returns a
value is the toString
method. This method is called automatically by Java in
a number of situations when it needs to convert an object to a String
. Most
notably the methods System.out.print
and System.out.println
use it to
convert a object argument into a String
to be printed and when objects are
added to String
s with +
and +=
their String
representation
comes from calling their toString
method.
Here is the Student
class again, but this time with a toString
method.
Note that when we call System.out.println(s1)
it will automatically call the
toString
method to get a String
representation of the Student
object. The toString
method will return a String
that is then printed out.
Watch how the control moves to the toString
method and then comes back to main
in the Java visualizer by using the Show CodeLens button.
See the toString() method in action.
5.4.2.
Programming Challenge : Class Pet¶
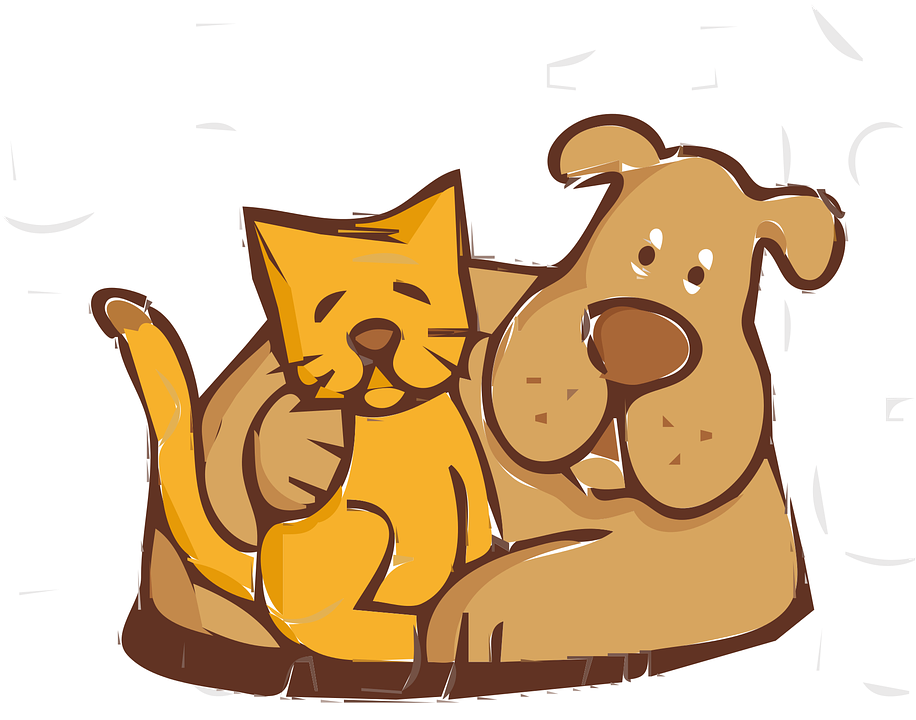
You’ve been hired to create a software system for the Awesome Animal Clinic! They would like to keep track of their animal patients. Here are some attributes of the pets that they would like to track:
Name
Age
Weight
Type (dog, cat, lizard, etc.)
Breed
Create a class that keeps track of the attributes above for pet records at the animal clinic. Decide what instance variables are needed and their data types. Make sure you use
int
,double
, andString
data types. Make the instance variablesprivate
.Create a constructor with many parameters to initialize all the instance variables.
Create getters for each of the instance variables.
Create a
toString
method that returns all the information in aPet
.In the
main
method below, create 2Pet
objects with different values and call the constructor, accessor methods, andtoString
methods to test all your code.Make sure you use good commenting!
Create a Pet class that keeps track of the name, age, weight, type of animal, and breed for records at an animal clinic.
5.4.3. Summary¶
A getter allows other objects to obtain the value of instance variables or static variables.
A non-void method returns a single value. Its header includes the return type in place of the keyword void.
A getter is a non-void method that returns the value of an instance variable. Its return type matches the type of the instance variable.
Methods “return by value” where a copy of the value is returned. When the value is a primitive type, the value is copied. When the value is a reference to an object, the reference is copied, not the object.
The
return
keyword is used to return the flow of control to the point immediately following where the method or constructor was called.The
toString
method is an overridden method that is included in classes to provide a description of a specific object. It generally includes what values are stored in the instance data of the object.If
System.out.print
orSystem.out.println
is passed an object, that object’stoString
method is called, and the returnedString
is printed.An object’s
toString
method is also used to get theString
representation used when concatenating the object to aString
with the+
operator.
5.4.4. AP Practice¶
- The getNumOfPeople method should be declared as public.
- Correct, accessor methods should be public so they can be accessed from outside the class.
- The return type of the getNumOfPeople method should be void.
- The method return type should stay as int.
- The getNumOfPeople method should have at least one parameter.
- This method should not have any parameters
- The variable numOfPeople is not declared inside the getNumOfPeople method.
- This is an instance variable and should be declared outside.
- The instance variable num should be returned instead of numOfPeople, which is local to the constructor.
- The numOfPeople variable is correctly returned.
5-4-4: Consider the following Party class. The getNumOfPeople method is intended to allow methods in other classes to access a Party object’s numOfPeople instance variable value; however, it does not work as intended. Which of the following best explains why the getNumOfPeople method does NOT work as intended?
public class Party { private int numOfPeople; public Party(int num) { numOfPeople = num; } private int getNumOfPeople() { return numOfPeople; } }
The id instance variable should be public.
-
Instance variables should be private.
The getId method should be declared as private.
-
Accessor methods should be public methods.
The getId method requires a parameter.
-
Accessor methods usually do not require parameters.
The return type of the getId method needs to be defined as void.
-
void is not the correct return type.
The return type of the getId method needs to be defined as int.
-
Correct! Accessor methods have a return type of the instance variable they are returning.
5-4-5: Consider the following class definition. The class does not compile.
public class Student
{
private int id;
public getId()
{
return id;
}
// Constructor not shown
}
The accessor method getId is intended to return the id of a Student object. Which of the following best explains why the class does not compile?