13.3. DecksΒΆ
In the previous chapter, we worked with a vector of objects, but I also
mentioned that it is possible to have an object that contains a vector
as an instance variable. In this chapter I am going to create a new
object, called a Deck
, that contains a vector of Card
s.
The structure definition looks like this
struct Deck {
vector<Card> cards;
Deck (int n);
};
Deck::Deck (int size) {
vector<Card> temp (size);
cards = temp;
}
The name of the instance variable is cards
to help distinguish the
Deck
object from the vector of Card
s that it contains.
For now there is only one constructor. It creates a local variable named
temp
, which it initializes by invoking the constructor for the
vector
class, passing the size as a parameter. Then it copies the
vector from temp
into the instance variable cards
.
Now we can create a deck of cards like this:
Deck deck (52);
Here is a state diagram showing what a Deck
object looks like:
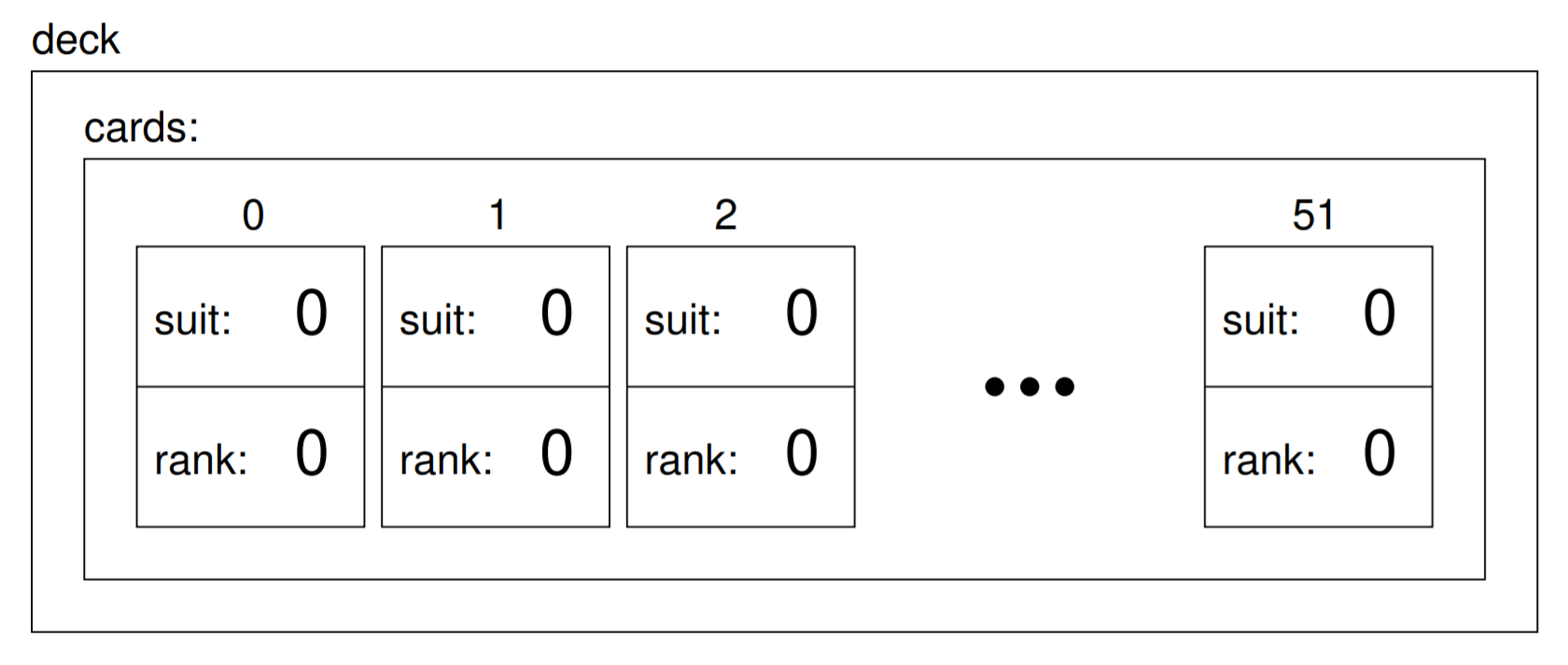
The object named deck
has a single instance variable named
cards
, which is a vector of Card
objects. To access the cards in
a deck we have to compose the syntax for accessing an instance variable
and the syntax for selecting an element from an array. For example, the
expression deck.cards[i]
is the ith card in the deck, and
deck.cards[i].suit
is its suit. The following loop
for (int i = 0; i<52; i++) {
deck.cards[i].print();
}
demonstrates how to traverse the deck and output each card.
- The ranks and suits of the cards are initialized to the proper ranks and suits in a standard deck of cards.
- Unless you the programmer tell it to, the computer won't do it.
- There will be 52 cards.
- We initialized cards with a value of 52.
- The ranks and suits will be initialized to their default values.
- In our case is, the default values are zero.
- The only instance variable in the deck is cards.
- cards is a vector of Cards!
- You can't access individual cards in the deck.
- You can access any card by indexing, for example: deck.cards[n].
Q-2: Take a look at the state diagram above. When we create a deck of cards using Deck deck (52)
,
what is true about our new deck?
- True - because this is the default mapping of enumerated types.
- The default mapping begins with 0.
- True - because our definition of rank overrides the default mapping.
- Our definition doesn't use the default mapping, which begins with 0.
- False - because this is the default mapping of enumerated types.
- The default mapping begins with 0.
- False - because our definition of rank overrides the default mapping.
- If we wanted to, we could have set the rank of ace to 7, and the rest of the cards would still be ranked in order.
Q-3: ACE
corresponds to a rank of value 0
.