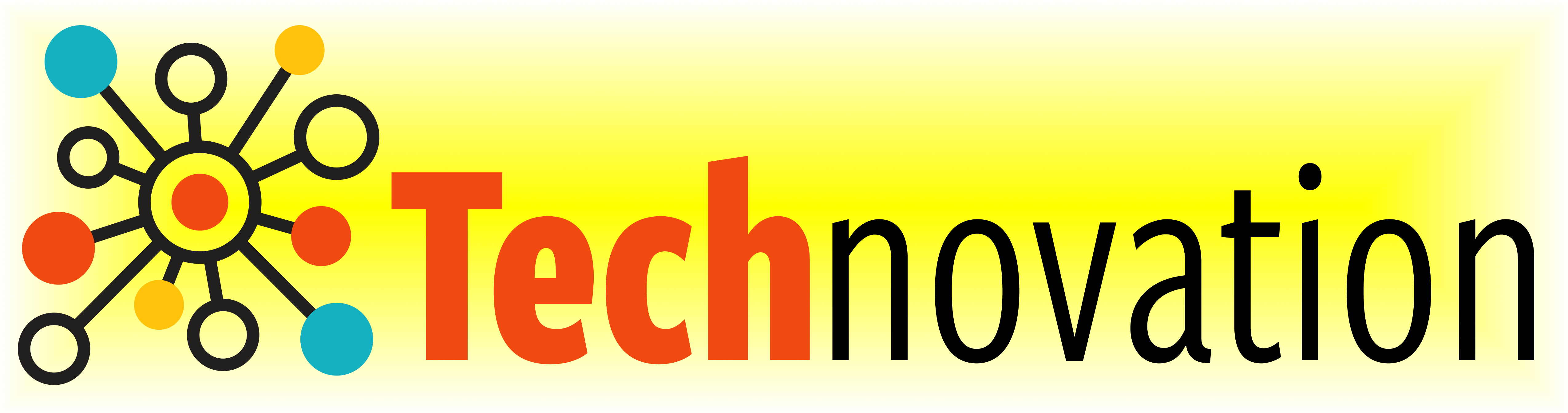
7.4. Practice Makes Perfect¶
Drawing commands |
What does it do? |
---|---|
|
Move forward a specified distance |
|
Move backward a specified distance |
|
Turns 90 degrees to the left (you can use any angle, not just 90!) |
|
Turns 90 degrees to the right |
|
Draws a circle with the specified radius |
|
Move straight to the position with coordinates (x, y). Note: the center is (0, 0) |
|
Stop leaving a trail |
|
Start drawing a trail |
|
Set the color to c (https://trinket.io/docs/colors) |
|
Starts filling in drawn shapes |
|
Stops filling in drawn shapes |
|
Determines how quickly the turtle will move through commands |
Drawing commands |
What does it do? |
---|---|
|
Repeat n times |
|
Creates and defines a new function |
|
Calls a function |
Command |
What does it do? |
---|---|
|
Stores the value in your variable |
|
Takes user input and stores it in your variable |
7.4.1. Quick review of variables:¶
A variable is a container that stores a value
You can access that value by calling your variable by its name
You can change the value that’s stored in a variable with the equal sign “=”
7.4.2. Parameters¶
turtle.circle(50)
we give it a value for the radius of the circle.
We can do this when we define our own functions too.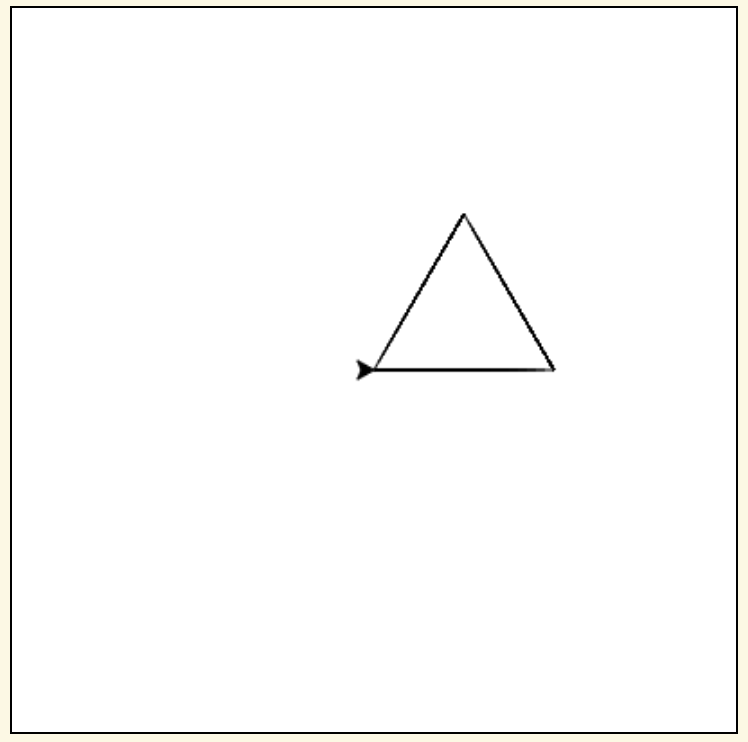
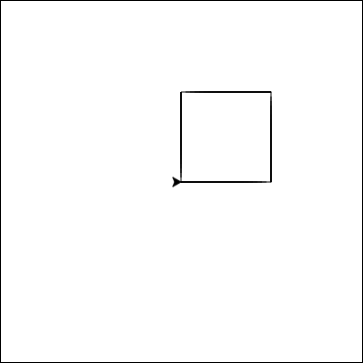
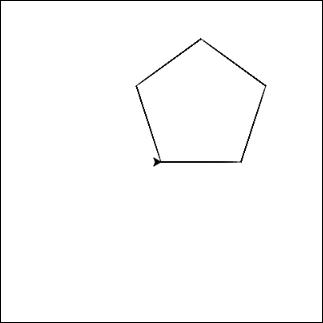
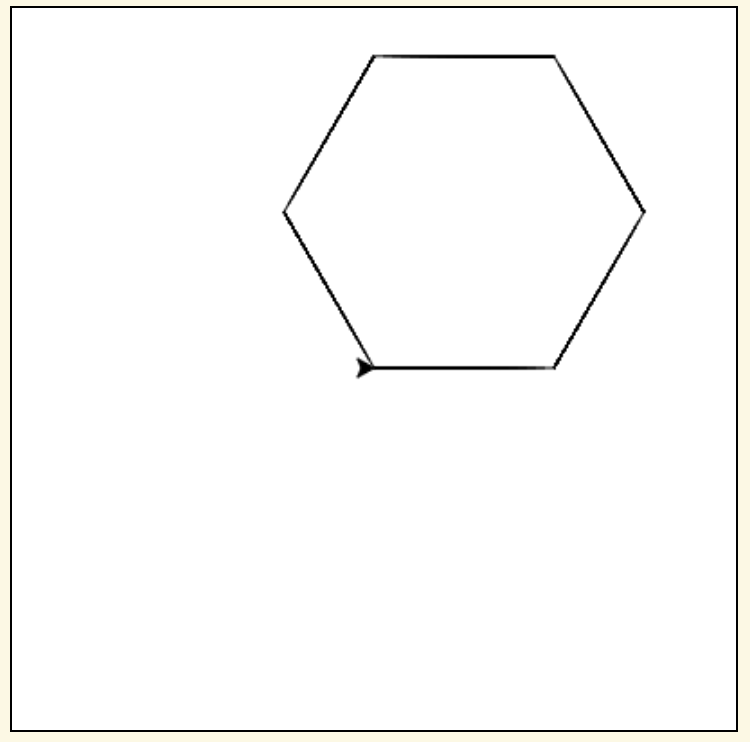
shape()
function that
has a parameter for the number of sides for the shape. (So shape(3)
draws a triangle, shape(4)
draws a square, etc)7.4.3. Have Some Fun!¶
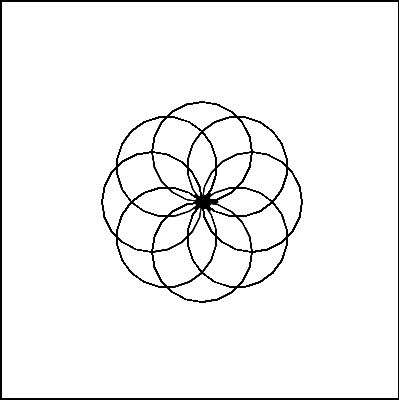
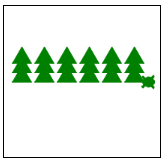
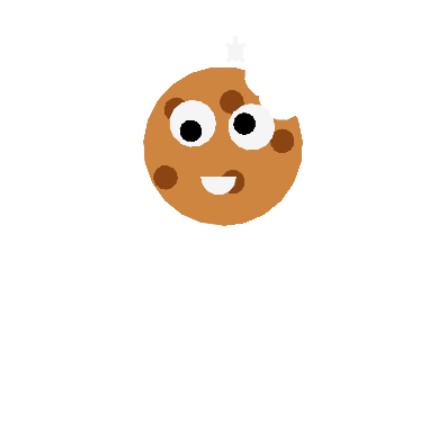
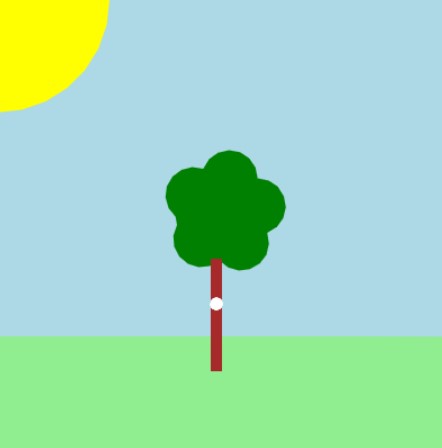
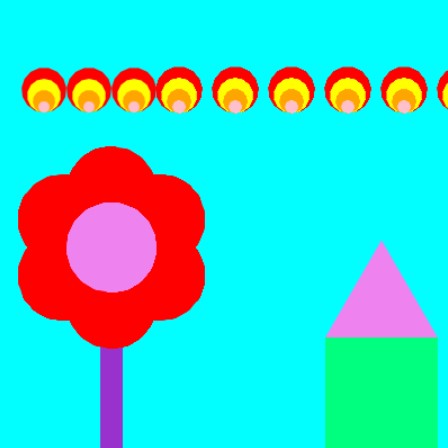
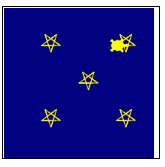
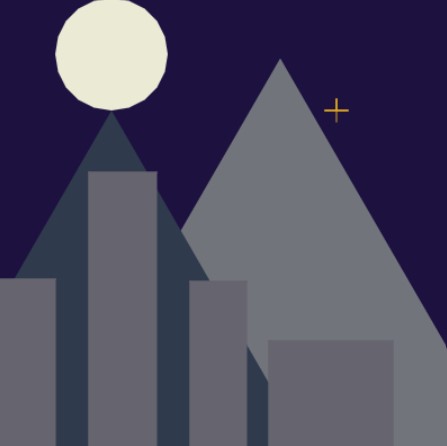