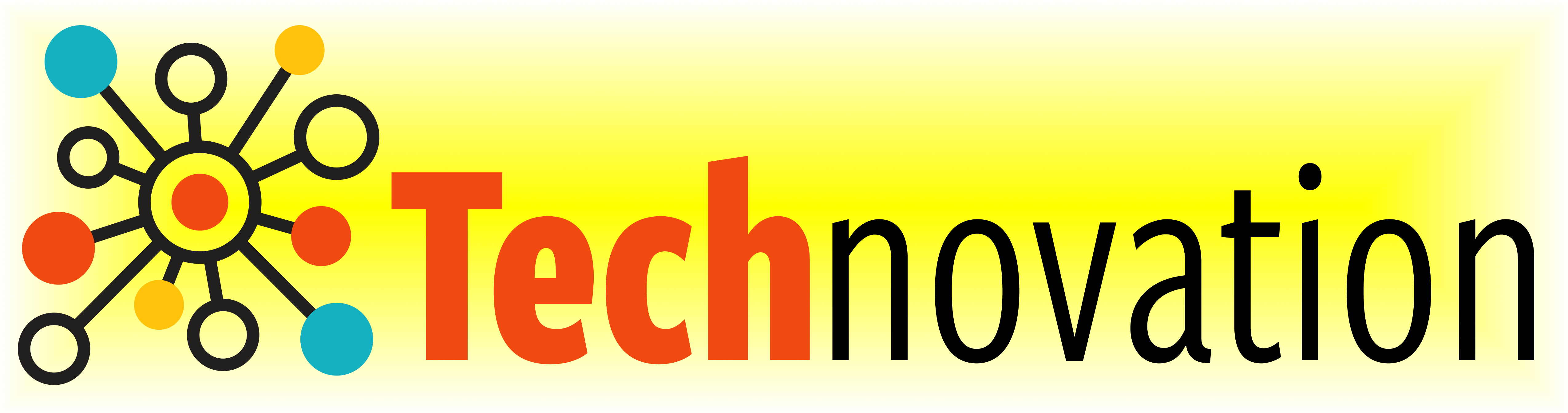
2.4. Learning to Code¶
2.4.1. Python Turtle
¶
Think of a Python turtle
as your new (virtual) pet.
Instead of using words and gestures
to teach it to roll over or sit, you write computer programs containing instructions
that tell it how to draw pictures on a screen.
For example, the code in the box below instructs the turtle
to draw a square.
Press the Run
button. You may need to scroll the window to see
the screen below the editor window.
1 # a square with side-length 100 pixels
A lines that start with a #
is a comments. The computer ignores all comments.
You write comments to help someone reading the code understand what the code does.
This comment tells the reader that the program creates a square measuring 100 pixels on each side.
2 import turtle
Imports the turtle
module that comes with Python. A module defines
one or more data objects and instructions.
Importing the module allows you to use these data objects and instructions
in your own program.
4 turtle.forward(100)
Moves the turtle
forward (i.e., in whatever direction it is facing)
by 100 pixels.
The 100
is an input to the instruction—the computer
reads this number to know how many pixels to move forward. Since the turtle
is facing right, this instruction will move it 100 pixels to the right.
5 turtle.left(90)
Rotates the turtle
90
degrees to the left (counter-clockwise).
The left
instruction needs one input—the computer reads the input (90
in
this instruction) to know how many degrees to rotate through.
The final two instructions are repeated three times to complete the other three sides of the square.
Notice that:
After the line 5
turtle.left(90)
instruction, theturtle
is facing up. So the line 6turtle.forward(100)
instruction moves it up by 100 pixels.The line 7
turtle.left(90)
then rotates theturtle
so it faces left and so the line 8turtle.forward(100)
instruction moves theturtle
left by 100 pixels.The line 9
turtle.left(90)
then rotates theturtle
so it faces down and so the line 10turtle.forward(100)
instruction moves theturtle
back down to where it started.Finally, the line 11
turtle.left(90)
instruction rotates theturtle
back to the start position—making it again face to the right.
Check your understanding of this program.
-
Q-2: Match each line from the Square Program (above) with the effect that executing it has
on the turtle.
(Line numbers are shown on the left.)
- 2 import turtle
- allows you to use data objects and instructions from the turtle module
- 4 turtle.forward(100)
- moves the turtle 100 pixels to the right
- 8 turtle.forward(100)
- moves the turtle 100 pixels to the left
- 9 turtle.left(90)
- makes the turtle face down
- 11 turtle.left(90)
- makes the turtle face to the right
- 1 # a square with side-length 100 pixels
- does not move or turn the turtle
- 6 turtle.forward(100)
- moves the turtle 100 pixels up
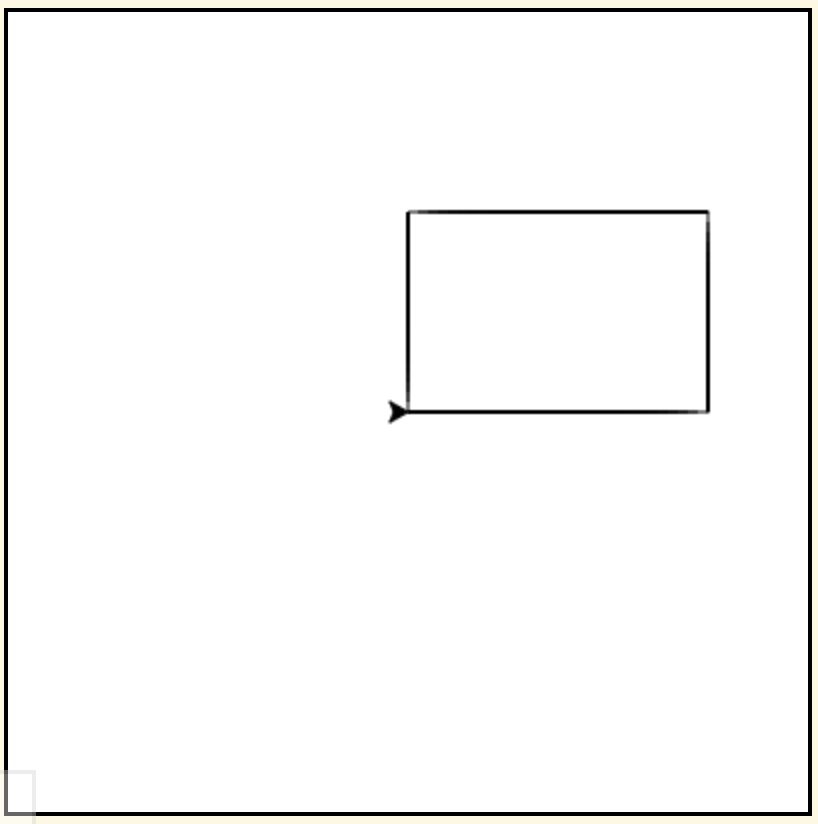
Rectangle Image¶
Arrange the instructions into a program that draws a rectangle 150 pixels wide and 100 pixels high, like the Rectangle Image shown above.
You can instruct a (Python) turtle
to do much more than just to move forward
and turn left
.
Following are some other instructions that you can give it.
See if you can guess what each does. Then press the button to check if you guessed correctly.
Instructs the turtle
to move L
pixels backwards
(opposite to the direction that the turtle is facing).
The input, L
, tells the computer how many pixels to move.
Instructs the turtle
to rotate D
degrees towards the right
(clockwise).
The input, D
, tells the computer how many degrees to rotate through.
Instructs the turtle
to go (straight) to the position with coordinates (X, Y)
.
The center of the screen is position (0, 0)
and units are measured in pixels.
The default screen-size in an active code widget is 400 pixels wide and 400 pixels high.
The inputs, X
and Y
, tell the computer what position to move to.
Instructs the turtle
to draw a circle with a radius of R
pixels long.
The turtle
draws the circle starting at its current location
and curving left from the direction of travel (the direction the turtle is
facing).
The input, R
, tells the computer how long to make the circle’s radius.
Instructs the turtle
to use the color C
for drawing.
By default, the initial turtle
color is black
.
The input, C
, tells the computer what color to use.
Instructs the turtle
to stop drawing as it moves.
Why “up”?
Think of attaching a felt-tip marker to the tail of the turtle
so that,
when the turtle
’s’ tail is up, it moves without making any mark and, when its tail is down,
it makes a solid line as it moves.
The turtle
starts with its tail down. So if you want to move it
without drawing anything, you have to instruct it
to lift it’s tail up (execute turtle.up()
) before you instruct it to move.
Instructs the turtle
to draw as it moves.
After executing a turtle.up()
instruction, if you want the
turtle
to start drawing again, you have
to execute a turtle.down()
instruction.
Instructs the computer to fill the figure drawn by executing the code between
the turtle.begin_fill()
and turtle.end_fill()
instructions.
- top left
- Correct! The turtle starts drawing at (-100, 0), goes up to (-100,100), then right to (100,100), and then down to (100,0), forming three sides of a rectangle; filling the shape creates the top-left image, with the turtle still at (100,0) and facing right.
- top right
- No. Trace the path that the turtle makes on paper. Then color every point that lies between two points on this path.
- bottom left
- No. Trace the path that the turtle makes on paper. Then color every point that lies between two points on this path.
- bottom right
- No. Trace the path that the turtle makes on paper. Then color every point that lies between two points on this path.
Q-4: Pretend to be a turtle
and follow the instructions in the next
program. (You might want to do it on a graph paper.)
Which of the shapes shown below will the program draw?
import turtle
turtle.up()
turtle.goto(-100, 0)
turtle.down()
turtle.color("lightblue")
turtle.begin_fill()
turtle.goto(-100, 100)
turtle.goto(100, 100)
turtle.goto(100, 0)
turtle.end_fill()
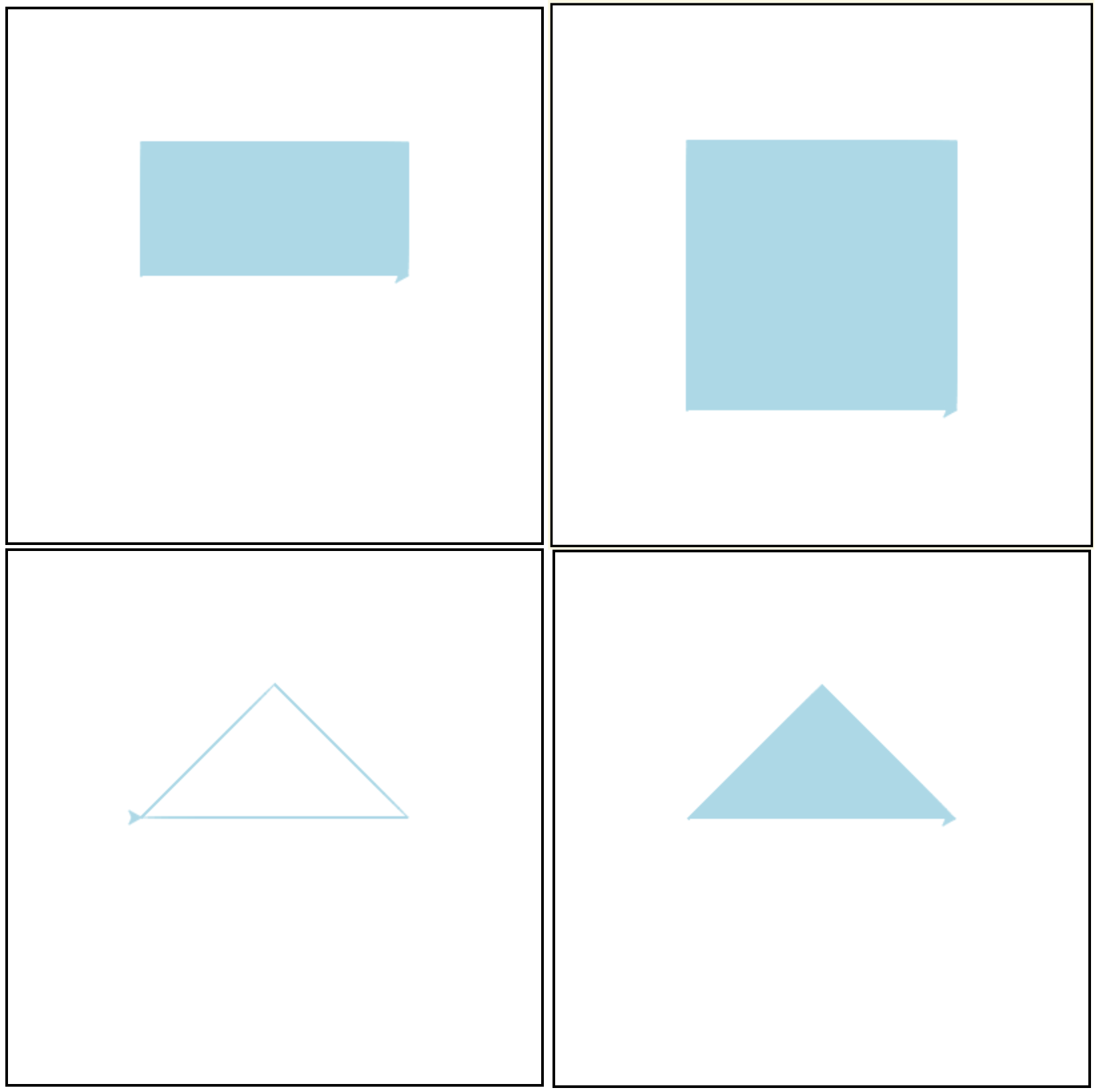
A Python Graphics turtle
is an example of what computer scientists
call a data object.
A data object is just a computer representation of something in
the application domain,
such as a number, a text, customer in an
airline reservation system, or a pen in a drawing program.
An important property of a data object is that it has a state.
The state of a data object affects what the object does when it
receives an instruction.
For example, the state of a turtle
determines whether it will draw a
line when it moves or not.
Before any turtle.up()
instructions, the turtle.forward(100)
instruction both draws a line
and changes the position of the turtle
;
but after a turtle.up()
instruction, a turtle.forward(100)
instruction only changes the turtle
’s
position. So executing turtle.up()
affects what occurs when
turtle.forward(100)
is executed.
Computer scientists refer to commands, like turtle.up()
and turtle.down()
, that change
the state of the data object that receives them as
having side effects.
turtle.forward(45) |
turtle.backward(90) |
turtle.left(30) |
turtle.right(90) |
turtle.color(“red”) |
turtle.circle(75) |
With these commands, we can instruct the turtle
to draw more interesting diagrams.
For example, here’s a program that draws a six-pointed star in two colors.
Run the program and scroll down to see what the turtle
draws.
The computer executes the instructions in a program exactly as they are
written and in the exact order. Your dog probably is not as obedient!
Sometimes the order doesn’t matter; other times it does.
1 import turtle & 4 turtle.up() |
4 turtle.up() & 5 turtle.goto(-100, -50) |
6 turtle.color(“green”) & 7 turtle.down() |
8 turtle.forward(200) & 9 turtle.left(120) |
16 turtle.left(60) & 17 turtle.up() |
6 turtle.color(“green”) & 19 turtle.color(“blue”) |
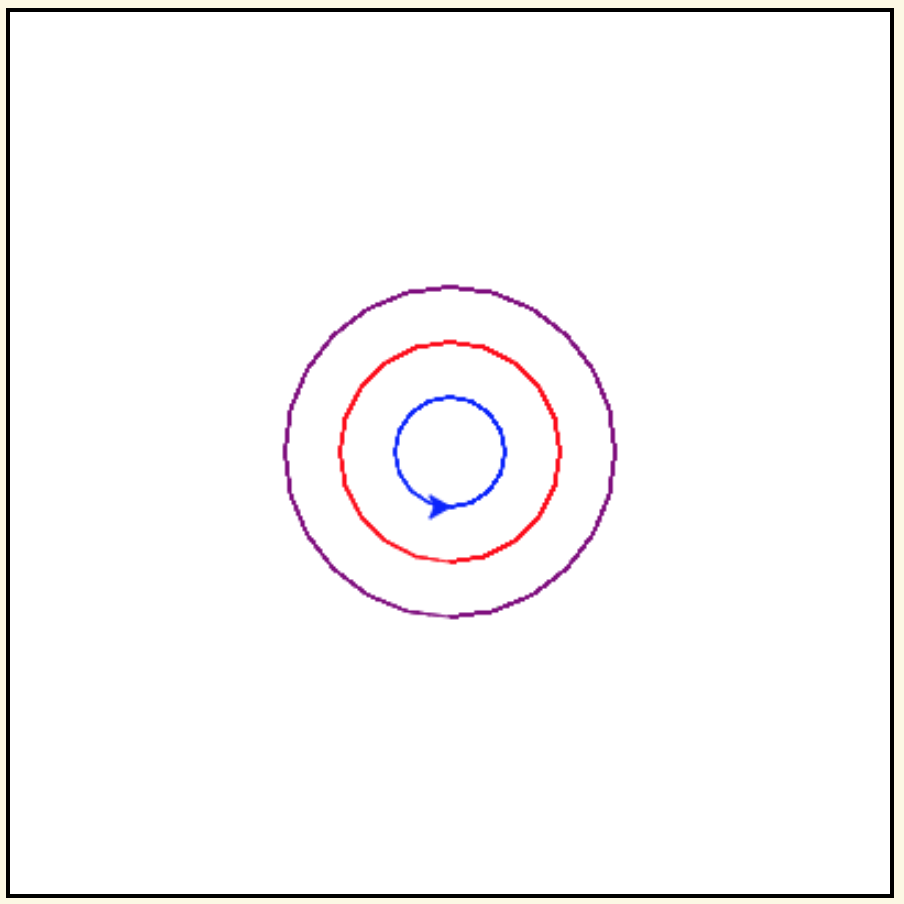
Concentric Circles Image¶
Arrange the instruction blocks below into a program that draws:
First, a purple circle of radius 75.
Then, a red circle of radius 50.
And finally, a blue circle of radius 25.
The drawing it produces should look like the Concentric Circles Image above.
(Drag the instruction blocks into the yellow rectangular region in the order that the computer should execute them.)
We’ll introduce more turtle
instructions as we go along.
But if you are curious, you can look here to learn all about Turtle Graphics,
including all of the instructions that the turtle
understands.
2.4.2. Python Basics¶
The History of Programming Languages (HOPL) listed 8,512 different programming languages in January of 2011! No doubt, there are even more by now!
The animation below gives you an idea of how the popularity of modern programming
languages has fluctuated in just the last 7 years. (With over 40 *million*
users and hosting more than 190 *trillion* public code bases,
hosts the largest collection of open-source software in the world.)
You might want to speed up this video before watching: Pause the video and
select the gear icon,
; then change the
playback speed
to 2.
You might think that becoming an expert programmer is hopeless since there are so many languages—how could you hope to learn even a small fraction of them? But the good news is that you don’t need to. Almost all current languages allow you to do the same basic things. So just pick one of them. We chose Python for this ebook since it is relatively easy to learn compared to some others and is as powerful as any. Also, most programming languages are based on the same basic concepts.
The rest of this section introduces four such concepts: Keywords, types, variables, and assignment. We will need these concepts to create more interesting drawings in later meetings.
2.4.2.1. Keywords
¶
All but the most primitive programming languages define words that mean something special to the computer. Called keywords, these words help the computer recognize the instructions that you want it to execute. There are 35 keywords in Python, as shown below.
Python Keywords |
||||
---|---|---|---|---|
False |
await |
else |
import |
pass |
None |
break |
except |
in |
raise |
True |
class |
finally |
is |
return |
and |
continue |
for |
lambda |
try |
as |
def |
from |
nonlocal |
while |
assert |
del |
global |
not |
with |
async |
elif |
if |
or |
yield |
Because these words already mean something to the computer, you can use them only in special instructions.
In later meetings, we’ll learn how to use many other keywords. For now, it’s enough to know that a keyword is a word that has a particular meaning in all programs.
2.4.2.2. Types
¶
A type is a set of values that share some common properties and/or operators.
Python has four built-in types; they are named: 1) int
, 2) float
, 3) bool
,
and 4) str
.
The first two are both numeric types.
An int
represents an integer (or a whole number).
The numeric values in our examples so far have all been int
’s.
In contrast, a float
represents a decimal number.
The computer figures out if a number is an int
or a float
by how you write it: a number with no decimal point is an int
and one containing a decimal point is a float
.
For example, 2
and -33
are int
’s and -2.0
and 1.414
are float
’s.
The numeric types share the usual arithmetic operators—addition (+
),
subtraction (-
), multiplication (*
),
division (/
), and so on—and also some more advanced operators,
which we probably won’t need to use in this ebook.
-100 |
1,000 |
100.0 |
55 |
-0.25 |
145 362 |
-100 |
1,000 |
100.0 |
55 |
-0.25 |
145 362 |
The bool
type has only two logical values: True
and False
.
In later weeks, we’ll be using logical values and operators
in instructions that require decision making.
Remember that Python is case-sensitive:
For example, true
is not the same as True
; and neither is TRUE
.
The term str
is short for string, which represents a series of characters (or text).
You write a string by placing single quotes ('
),
double quotes ("
), or triple double-quotes ("""
) around its characters.
The quotes that start and end a string have to match—in other
words, if you start a string
with a single quote, you have to end it with a single quote, and so on for
the other quotes.
For example,
'Hello World!'
"Hello World!"
and
"""Hello World!"""
all represent the same 12-character string (the space and exclamation point are both characters).
"It's a done deal." red '1,000' '-3' ".' 'I'm fine, thanks.' """True"""
2.4.2.3. Variables
¶
If you did the practice exercises from our last meeting, you probably got the feeling that variables are useful. If so, you are right! Almost all programming languages allow coders to create variables.
A variable is like a container that you have labeled with a name (the variable name). When the computer executes a program, you can instruct it to store a value in the variable (container) and then use that value in a later instruction.
In Python, the name that you give to a variable has to satisfy three rules:
It must consist of one or more lower case letters (
a
toz
), upper case letters (A
toZ
), digits (0
to9
), and/or underscores (_
).It cannot start with a digit.
It cannot be identical to a Python keyword.
item3 |
_radius |
Length |
A_2_Z |
item 3 |
guru |
import |
Import |
1_a |
true |
True_no |
_103 |
A good container label is one that reminds you what the container contains so
you don’t have open it to find out.
In much the same way, a good variable name is one that reminds you what
your code will use the variable for.
For example, you might name a variable radius
if it will store
the number of pixels in the radius of a circle or time_left
if it will
store the number of minutes left in a game.
Make the code easier to read |
Make the code execute faster |
Make the code easier to modify |
Make the logic easier to see |
Make the code more complex |
Make your co-coders happier |
2.4.2.4. Assignment
¶
The instruction for storing a value in a variable is called an assignment. In Python, an assignment has the form
var = exp
where var
is the name of a variable and exp
is an expression.
For example, y = x + 1
is an assignment.
The symbol =
is called the assignment operator.
An expression is like a recipe that a computer can evaluate (execute) to create a value.
Evaluation of the expression is said to return this value.
The simplest expressions are values from the built-in types,
like 5
, True
, -52.79
, or "Name"
, and variables.
Evaluation of a value just returns the value.
Evaluation of a variable returns the value stored in the variable;
so if x
stores the value 5
, then evaluation of x
returns 5
.
You can write more complex expressions by applying operators to expressions.
For example, x + 1
is an expression. When evaluated, it returns the value that is
one more than the value stored in x
.
So if x
stores 5
, evaluation of x + 1
returns 6
.
We won’t list all the Python operators since you can easily look them up when you need them. But you can click on ‘Some Numeric Operators’ to see examples of the operators we will use in later examples. You should recognize most of these operators from your studies of arithmetic, although some may have been written differently.
The following examples illustrate evaluation of some useful arithmetic operators.
+
(addition or plus): Returns an int
if both operands are int
’s; or
a float
if either operand is a float
.
5 + 12
returns17
1.0 + 3.0
returns4.0
7 + 1.2
returns8.2
-
(subtraction or minus): Same typing rules as for +
5 - 10
returns-5
5 - 10.0
returns-5.0
0.1 - 0.1
returns0.0
*
(multiplication): Same typing rules as for +
10 * 5
returns50
0.2 * 10
returns2.0
/
(division): Always returns a float
2 / 10
returns0.2
10 / 2
returns5.0
10.0 / 2.0
returns5.0
//
(quotient or integer division) and %
(remainder): Usually used
with int
operands, in which case both returns int
’s .
Since 5 can be subtracted from 13 a total of 2 times leaving a remainder of 3:
13 // 5
returns2
and13 % 5
returns3
Since 200 is too big to subtract from 109 (it can be subtracted only 0 times leaving a remainder of 109):
109 // 200
returns0
and109 % 200
returns109
An assignment instructs the computer to store the value returned by evaluating
the expression on the right of the =
in the variable on the left.
For example, when the value of x
is 5
, executing
y = x + 1
stores 6
(the value returned by evaluation x + 1
) in y
.
An experienced programmer will not read y = x + 1
as: “y equals x plus one”
Instead, they might read it as: “assign x plus one to y”
Or: “set y to x plus one”
Or: “y is assigned x plus one”
Or even: “y gets x plus one”
The code below is the beginning of a turtle graphics program that we’ll add to in the practice exercises for next week. See what you can understand of it by follow the instructions and calculating answers for the questions below.
1
2 import turtle
3
4
5 ext_length = 100
6 ext_height = 150
7 border_width = 20
8 border_color = "tan"
9 inter_color = "green"
10
11
12 inter_length = ext_length - (2 * border_width)
13 inter_height = ext_height - (2 * border_width)
14
15
16 inter_area = inter_length * inter_height
17 border_area = (ext_length * ext_height) - inter_area