Free Response - Delimiters B¶
Part B¶
(b) Write the method isBalanced
, which returns true
when the delimiters are balanced and returns false
otherwise. The delimiters are balanced when both
of the following conditions are satisfied; otherwise they are not balanced.
When traversing the
ArrayList
from the first element to the last element, there is no point at which there are more close delimiters than open delimiters at or before that point.the total number of open delimiters is equal to the total number of close delimiters.
Consider a Delimiters
object for which openDel` is "<sup>"
and closeDel
is "</sup>"
. The examples below show different ArrayList
objects that could
be returned by calls to getDelimitersList
and the value that would be returned by a call to isBalanced
.
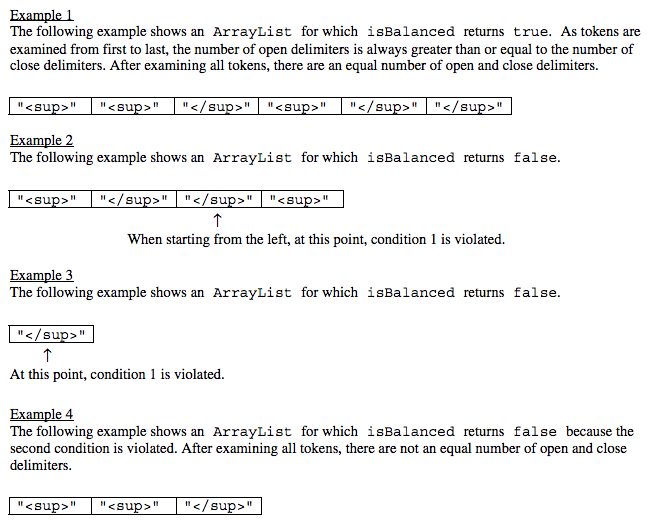
Check your understanding of the Question¶
The problems in this section can help you check your understanding of part B. You can skip these if you think you know what to do already. Click on a button to reveal a question.
- String
- What type are false and true?
- boolean
- The values false and true are of type boolean.
- int
- In some languages false and true are represented by integers, but not in Java.
- ArrayList
- What type are false and true?
8-24-10-1: What type does isBalanced return?
- openDel
- openDel holds the open delimiter
- closeDel
- closeDel holds the close delimiter
- Delimiters
- Delimiters is the class name
- delimiters
- delimiters is the variable which is passed to the isBalanced method
8-24-10-2: What is the name of the variable the code will be looping through?
How to Solve Part B¶
Here is the question again.
Write the method isBalanced
, which returns true
when the delimiters are balanced and returns false
otherwise. The delimiters are balanced when both
of the following conditions are satisfied; otherwise they are not balanced.
When traversing the
ArrayList<String> delimiters
from the first element to the last element, there is no point at which there are more close delimiters than open delimiters at or before that point.the total number of open delimiters is equal to the total number of close delimiters.
8-24-10-3: Explain in plain English what your code will have to do to answer this question. Use the names given above.
This section contains a plain English explanation of one way to solve this problem as well as problems that test your understanding of how to write the code to do those things. Click on a button to reveal the algorithm or problem.
The method isBalanced
will loop through delimiters
and keep track of the number of open and close delimiters we have found so far. To do that we can create two integer variables: totalOpen
and totalClose
and set them to 0
initially. Each time through the loop we will check if the current string which we will call currString is equal to openDel
and if so increment totalOpen
, otherwise if it is equal to closeDel
increment totalClose
. Next if totalClose
> totalOpen
the method should return false. A
After the loop return totalOpen
== totalClose
. This will return true if they are equal and false otherwise.
- while
- You can use a while loop, but it would make your code more error prone than another type of loop
- for
- You can use a for loop, but it would make your code more error prone than another type of loop
- for-each
- Since you need to loop through all the strings in the ArrayList in order, a for-each loop would be best
- nested for loop
- There is no need for a nested loop in this situation
8-24-10-4: Which loop would be best for this situation?
- if (currString = openDel)
- You must declare the type for delList
- if (currString == openDel)
- You must include the () when creating a new object
- if (currString.equals(openDel))
- You must create an ArrayList using a concrete subclass like ArrayList
- if (currString.equal(openDel))
- The declared type must be the same or a parent class of the actual type.
8-24-10-5: Which Java expression correctly tests if currString is equal to openDel?
Write the Code¶
Write the method isBalanced
, which returns true
when the delimiters are balanced and returns false
otherwise. The delimiters are balanced when both
of the following conditions are satisfied; otherwise they are not balanced.
When traversing the
ArrayList
from the first element to the last element, there is no point at which there are more close delimiters than open delimiters at or before that point.the total number of open delimiters is equal to the total number of close delimiters.
Write the method isBalanced
in the code below. The main
method contains code to test your solution.