Section 1: Generating Sentences¶
We have generated language in several ways in this class already.
One way was with the simple language generator. We typed in a model (a sentence pattern) and a dictionary of words by parts.
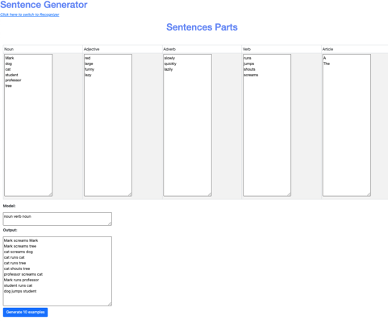
We have also done sentence generation in Snap. We have a language generation project that lets us generate sentences by parts, just like in the simple language generator.

The Python Version V1¶
The below program does the same thing in Python. Click ‘Run’ to generate a random sentence.
Try answering these questions about the code above.
- They are both variables. `nouns` is a list of nouns, and `noun` is a randomly chosen noun
- Yes, exactly right.
- Just the `s`. They mean the same thing.
- No, the names of the variables are just chosen to inform the reader.
- Both are variables, which hold data in Python
- That's true, but that doesn't get at the purpose of the words.
Q-2: What’s the difference between nouns and noun above?
- Tells Python that it is going to make a choice among these items
- No -- the choice comes later
- Creates a variable
- No, the variable name with `=` does that.
- Define a list, like our multiline or list blocks in Snap.
- That's right.
Q-3: What do you think the square brackets [] do in the above Python code?
- It generates a random number
- No -- the random library can generate a random number, but that's not what random means
- It's a fairly arbitrary variable name
- No, it's pre-defined in Python.
- It's the name of the library that contains the function `choice`
- That's right.
Q-4: Take a guess what the word random is above?
The Python Version V2¶
The below program does the same thing in Python. Click ‘Run’ to generate a random sentence.
Try answering these questions about the code above.
- A list of adjectives
- Not quite, 'adjectives' holds the list not 'adjective'.
- A randomly selected string from the 'adjectives' list.
- Yes, exactly right.
- A first string from the 'adjectives' list.
- Not always, it may return the first string, but it can also return any other string in the list.
Q-6: What does the variable ‘adjective’ hold in the above snippet?
- "A bee explores cautiously"
- Yes, this answer is missing an adjective.
- "The curious bird roams slowly"
- No, this answer contains all the required components.
- "A small bat flies urgently"
- No, this answer contains all the required components.
Q-7: Which of the following is a sentence that could NOT be produced from the code above?
- nouns = ["bee","bird","bat"]
- No,you will need to add "boat"
- verbs = ["flies", "explores", "roams"]
- No, you will need to add "floats"
- adjective = random.choice(adjectives)
- Yes, this line stays the same
- print(article,adjective,noun,verb,adverb)
- No, you will need to remove the final ",adverb"
Q-8: Let’s say that you want to make it possible for to generate “A curious boat floats.” Which of the lines below do you not have to change?
Section 2: Creating a little Python chatbot¶
You have built Chatbots in both Snap! and Charla-bots. Here’s an example on a little one:
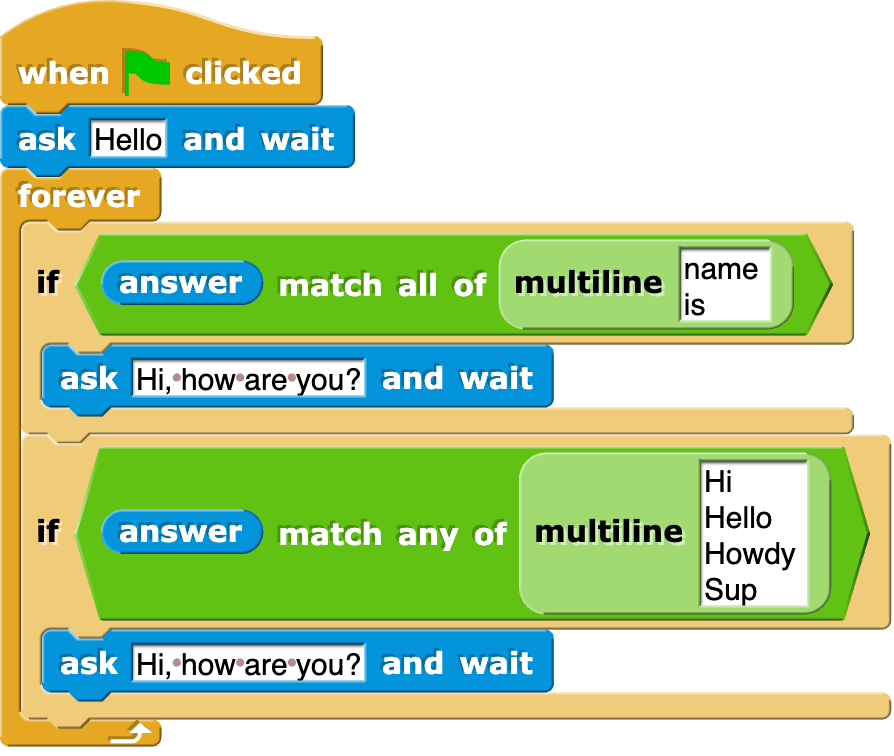
Here is a (very) little Python chatbot. This one is a little more sophisticated than our Snap chatbot – it can pick out a name from an input sentence, and it can do the equivalent of respond randomly that we saw in Charla-bot.
Python here in a Runestone ebook can’t receive user input, so let’s just change the inputSentence variable to represent what the user says. Press Run to see what the chat bot says.
This code is recognizing words in the inputSentence then deciding what to print in response.
inputSentence.split() breaks up the input into distinct words.
The first block that starts with if is asking if the input sentence contains the word “name”.
If the word “name” is there, the function index tells us where “name” appears. That goes in nameIndex.
If the word “is” is just after “name”, then we guess that the next word is the user’s name. Put that in yourName.
Then, print out a greeting for the specific name. (Remember that bots often focus on one word the user says.)
If the word “name” isn’t there, we check all the possible greetings words.
If one of those greeting words is in the input sentence, then print a basic “Hi, how are you?”
If we do find a greeting word, we set a variable that we found one. We break out of the loop checking the greeting words.
If we did not find a greeting word (not found), then we print a random question back to the user.
- Checks to see if the input sentence has the word "name" in it.
- Exactly. `wordsInSentence` is the list of words in the input sentence
- Puts the word "name" into the output
- No, output is generated with print()
- Asks the user what their name is
- No, nothing here does that.
Q-10: What do you think if “name” in wordsInSentence does?
- Lists the questions that the user might ask.
- No, that isn't happening here.
- Provide possible responses like *respond randomly* in Charla-bots.
- Exactly. Each question is a like another line in *respond randomly*.
- Makes it possible for the computer to respond to questions.
- No, those aren't questions that the computer can respond to.
Q-11: What do you think the variable questions is doing?
- How are you?
- No, that doesn't contain any of the words in `greetings`
- Hey, Sup
- Yes, because "Sup" is in `greetings`.
- Hola, Dude
- No, that sentence doesn't contain any of the words in `greetings`
Q-12: Which of these inputSentence options (and you’re welcome to try them!) would generate the chatbot saying “Hi, how are you?”
- True
- False
Q-13: found in this program is just a variable, that could be named anything, but it’s purpose is to track if we found a greeting word.